The realm of deep learning has witnessed a surge in popularity, fueled by its remarkable success in solving complex problems across various domains. From image recognition and natural language processing to self-driving cars and drug discovery, deep learning is transforming industries at an unprecedented pace. One of the key frameworks empowering this revolution is PyTorch, a highly flexible and powerful library developed by Facebook. For those seeking to streamline their deep learning workflows and enhance model development efficiency, PyTorch Lightning emerges as a game-changer. This comprehensive guide will delve into the intricacies of deep learning with PyTorch Lightning, equipping you with the knowledge and resources to embark on your own journey of cutting-edge AI development.
Image: github.com
Imagine a world where your deep learning models can be trained and deployed with unparalleled ease, where the complexity of managing multiple training loops and hyperparameters fades into the background, replaced by a streamlined and intuitive interface. This is the promise of PyTorch Lightning, a lightweight and high-performance wrapper that simplifies the process of training deep neural networks, allowing you to focus on the core aspects of your models, such as architecture design and hyperparameter optimization.
Why PyTorch Lightning? Unveiling the Advantages
PyTorch Lightning is not merely a library but a paradigm shift in how deep learning models are developed and managed. Its core philosophy revolves around code organization, efficiency, and scalability. By abstracting away the boilerplate code associated with training loops, data loading, and optimization procedures, PyTorch Lightning empowers you to achieve the following:
- Enhanced Code Readability and Organization: PyTorch Lightning’s modular structure promotes clean code organization, making your models easier to understand, maintain, and collaborate on.
- Reduced Boilerplate Code: PyTorch Lightning handles the complexities of training loops, data loading, and optimization, freeing you to focus on model architecture and hyperparameter tuning.
- Simplified Experiment Tracking: PyTorch Lightning seamlessly integrates with experiment tracking tools like Weights & Biases, enabling you to monitor training progress, log metrics, and visualize results effortlessly.
- Seamless GPU and TPU Integration: PyTorch Lightning leverages the power of GPUs and TPUs to accelerate training and inference, making it ideal for large-scale deep learning projects.
- Scalability and Flexibility: PyTorch Lightning supports distributed training, allowing you to train models on multiple GPUs or TPUs for faster convergence and improved performance.
Navigating the Landscape: Understanding PyTorch Lightning
1. Core Components: Defining the Building Blocks
PyTorch Lightning is built upon a few key components, each serving a specific purpose. Let’s explore these building blocks and understand how they work together to create a robust deep learning pipeline:
- LightningModule: The cornerstone of PyTorch Lightning, the LightningModule class encapsulates the essential functions of your deep learning model, including forward pass, loss calculation, and optimization steps. By subclassing LightningModule, you inherit a streamlined structure that facilitates clean code organization and efficient training.
- Trainers: PyTorch Lightning’s Trainer class orchestrates the entire training process. It manages data loading, model optimization, and progress tracking, allowing you to customize training parameters and configure hardware resources.
- Data Modules: The Data Module class is responsible for handling data loading and preprocessing. It encapsulates data transformations, batching, and shuffling, ensuring efficient and consistent data handling during training.
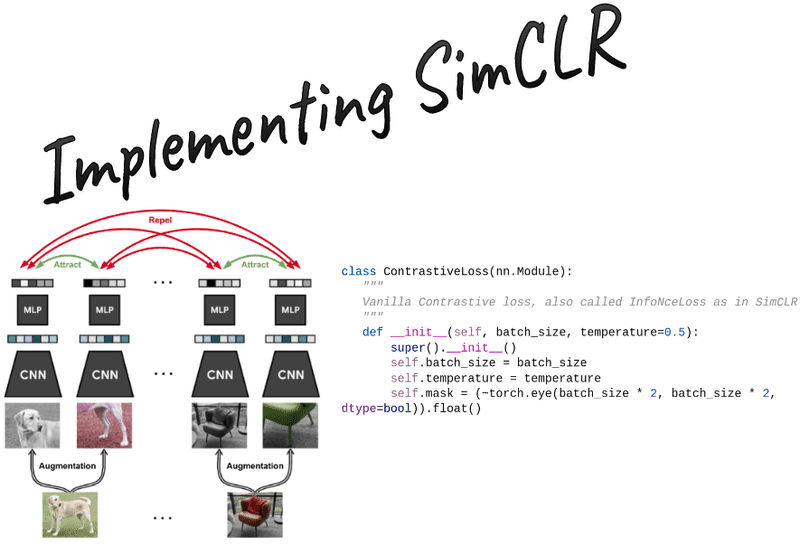
Image: theaisummer.com
2. Training with PyTorch Lightning: A Practical Walkthrough
Now, let’s dive into a practical example to demonstrate the simplicity and elegance of training a deep learning model using PyTorch Lightning. Consider a basic image classification task where you aim to classify images into different categories. With PyTorch Lightning, the training workflow is remarkably straightforward:
Step | Description | Code Snippet |
---|---|---|
1. Import Libraries | Import the necessary libraries: PyTorch Lightning, PyTorch, and any additional modules for data processing or visualization. |
|
2. Define the Model (LightningModule) | Create a subclass of pl.LightningModule and implement the necessary methods: forward (for forward pass), configure_optimizers (for setting up the optimizer), and training_step (for defining the training loop). |
|
3. Create Data Modules (Optional) | Optionally, create a subclass of pl.LightningDataModule to manage data loading, preprocessing, and batching. |
|
4. Initialize the Trainer | Create an instance of the pl.Trainer class and configure training parameters, such as number of epochs, learning rate, and device (CPU or GPU). |
|
5. Train the Model | Initiate the training process by calling the fit method of the Trainer object, passing the model and the data module (if defined). |
|
3. Beyond the Basics: Exploring Advanced Features
PyTorch Lightning’s capabilities extend far beyond the fundamental training workflow outlined above. Let’s delve into some of its advanced features that empower you to tackle more complex deep learning challenges:
- Hyperparameter Optimization: PyTorch Lightning facilitates easy integration with hyperparameter optimization libraries like Optuna and Ray Tune, enabling you to discover optimal model configurations.
- Distributed Training: PyTorch Lightning seamlessly supports distributed training, allowing you to leverage the computational power of multiple GPUs or TPUs for faster model convergence.
- Early Stopping: PyTorch Lightning provides built-in support for early stopping, which prevents the model from overfitting by monitoring the validation loss and automatically halting training when performance plateaus.
- Callbacks: PyTorch Lightning allows you to customize the training process with callbacks, which are functions that execute at specific points during training, such as epoch completion or validation steps.
Resources and Recommendations: Deep Dive into PyTorch Lightning
For those seeking to delve deeper into the nuances of PyTorch Lightning and explore its potential to revolutionize your deep learning workflows, here are some valuable resources and recommendations:
- Official Documentation: The official PyTorch Lightning documentation is an excellent starting point, providing comprehensive information on all aspects of the framework, including installation, usage, and advanced features. (https://pytorch-lightning.readthedocs.io/en/latest/)
- GitHub Repository: Explore the PyTorch Lightning GitHub repository for code examples, tutorials, and community discussions. Contribute to the open-source community by submitting bug reports and feature requests. (https://github.com/Lightning-AI/pytorch-lightning)
- Community Forum: Engages with the vibrant PyTorch Lightning community through the official forum, where you can pose questions, share insights, and seek assistance. (https://discuss.pytorchlightning.ai/)
- Blog Posts and Tutorials: Numerous blog posts and tutorials are available online, covering various aspects of PyTorch Lightning, from basic usage to advanced techniques.
Tips and Expert Advice: Unleashing the Power of PyTorch Lightning
Drawing upon my experience in deep learning, here are some practical tips and insights that can enhance your journey with PyTorch Lightning:
- Start Small: Begin with simple examples and gradually increase complexity. This approach fosters a gradual understanding of the framework and allows you to build confidence in your abilities.
- Utilize Callbacks: Embrace the power of Callbacks to customize and optimize your training process. Implement callbacks for tasks such as saving checkpoints, logging metrics, or performing early stopping.
- Experiment Tracking: Embrace the benefits of experiment tracking tools like Weights & Biases to monitor training progress, log metrics, and visualize results. This practice allows for systematic exploration of hyperparameters and model configurations.
- Leverage Distributed Training: Utilize PyTorch Lightning’s support for distributed training to accelerate model training, especially when dealing with large datasets or complex models.
- Engage with the Community: Join the PyTorch Lightning community, ask questions, share your experiences, and collaborate with fellow deep learning enthusiasts. This collective learning environment can accelerate your understanding and problem-solving capabilities.
FAQs: Addressing Common Questions
1. What are the main advantages of using PyTorch Lightning over pure PyTorch?
PyTorch Lightning offers numerous advantages, including streamlined code organization, reduced boilerplate code, simplified experiment tracking, seamless GPU and TPU integration, and advanced features like hyperparameter optimization and distributed training.
2. Is PyTorch Lightning suitable for beginners in deep learning?
Absolutely! While some familiarity with PyTorch is helpful, PyTorch Lightning’s intuitive design makes it accessible to beginners as well. Its modular structure and abstraction of complex tasks allow you to focus on the core concepts of deep learning.
3. Can I use PyTorch Lightning for NLP tasks?
Yes, you can. PyTorch Lightning is a versatile framework and can be employed for various deep learning tasks, including natural language processing. The LightningModule class allows you to implement models specific to NLP tasks, such as sequence-to-sequence modeling or text classification.
4. How can I access the PDF resource mentioned in the title?
To further your understanding and exploration, you can access a companion PDF resource by visiting the official PyTorch Lightning website. It provides comprehensive tutorials, practical examples, and insights into the latest advancements.
Deep Learning With Pytorch Lightning Pdf
Conclusion: Embarking on your Deep Learning Journey with PyTorch Lightning
PyTorch Lightning is a powerful and versatile framework that empowers deep learning enthusiasts and professionals alike. Its streamlined structure, efficient execution, and seamless integration with various tools and platforms make it an indispensable tool for modern AI development. As you delve deeper into the world of deep learning, embrace the simplicity and efficiency of PyTorch Lightning to make your model development journey more rewarding and fruitful.
Are you interested in learning more about PyTorch Lightning and its applications in deep learning?